Glossary groups
General API terms
These terms are all related to APIs in general.
- API (Application Programming Interface)
- A set of definitions, protocols, and tools for building software applications. APIs allow different systems to interact with each other programmatically. This course focuses mostly on REST APIs, but there are also language-based APIs such as Java, C++, Python, and more. I refer to these as native library APIs.
- API contract
- A documented agreement that specifies the behavior of the API, including endpoints, request methods, request payloads, response schemas, and more. See also OpenAPI specification document.
- API documentation
- Documentation that describes how an API works so that developers can understand and use it. Usually includes reference documentation, tutorials, code samples, and overviews.
- API gateway
- A server that acts as an intermediary for requests, often providing features like rate limiting, logging, security measures, and more. Many modern API gateways offer built-in support for importing Swagger (OpenAPI) definitions. This allows developers to quickly deploy, manage, and monitor their APIs using the Swagger definition as a starting point.”
- API key
- A unique identifier required to authenticate API requests. API keys control access to the API.
- API portal
- A developer-facing website that provides documentation, support, and access management for an API. Serves as the main interface for API consumers.
- API reference
- The documentation describing the endpoints, requests, parameters, responses, schemas, and other details about an API.
- API versioning
- A technique to make changes to your API without breaking the contract for existing users.
- authentication
- The process of verifying the identity of a user or client making an API request. Common authentication methods include API keys, OAuth, and basic auth.
- authorization
- The process of determining what permissions an authenticated user has for different operations and resources in the API.
- curl
- A command line tool for transferring data with URL syntax. curl is commonly used to demonstrate example API requests.
- developer portal
- A website that brings together documentation, code samples, SDKs, tools, and other resources to help developers use an API or set of APIs. The portal is a central location for developers to access everything they need.
- endpoint
- The specific address (URL) where an API can be accessed. It’s combined with the HTTP method to define the operations available. The endpoints indicate how you access the resource, while the method indicates the allowed interactions (such as GET, POST, or DELETE) with the resource. The same resource usually has a variety of related endpoints, each with different paths and methods but returning different information about the same resource. Similar to base url.
- GraphQL
- A query language and runtime for APIs, allowing clients to request only the data they need.
- HATEOAS
- Stands for Hypermedia as the Engine of Application State. Hypermedia is one of the characteristics of REST that is often overlooked or missing from REST APIs. In API responses, responses that span multiple pages should provide links for users to page to the other items.
- native library API
- API with code libraries for incorporating functionality directly into an application, rather than using network calls. Tied to a language and usually has a library file that you integrate into your project. Also called library API or class-based API.
- parameters
- Options that can be passed with an endpoint to influence the response, such as specifying the response format or number of results returned. Common types are header, path, and query string parameters.
- paths
- The available endpoints and operations in an API. Paths are the core resources that make up an API’s interface.
- rate limiting
- Policies that restrict the number of requests a user can make to prevent overload or abuse. Requests over the limit may be throttled or blocked.
- request
- A call made to an API, including the endpoint URL and parameters, headers, authorization, and other components needed to retrieve the desired information.
- response
- The data an API returns after receiving and processing a request. The response contains requested information or confirmation that an operation succeeded.
- response body
- The data returned by the server in response to a client’s request. It can contain information like resources (in case of a GET request) or status messages (like error messages). Usually in structured formats such as JSON or XML.
- REST API
- An API that follows REST (Representational State Transfer) principles by exposing resources through endpoints that can be interacted with using standard HTTP methods like GET, POST, PUT, and DELETE. REST APIs return data in easy-to-process formats like JSON.
- resource
- The core object or information managed by the API. Resources have different representations that can be retrieved or manipulated. A resource can be a single entity or a collection. For example, ‘users’ might represent a collection of users, while users/1’ represents a single user entity.
- SDK (Software Development Kit)
- A collection of tools and resources that allows developers to program applications for a specific platform, software, or service. SDKs include API client libraries, documentation, code samples, and guides. Note that an SDK can include various APIs, but an API does not include an SDK.
- SOAP (Simple Object Access Protocol)
- A messaging protocol for communication between web services, a predecessor to REST in many contexts.
- specification
- A detailed technical description of an API’s architecture, endpoints, parameters, sample requests/responses, and other implementation details. The most common API specification format is OpenAPI.
- Swagger
- A framework for the OpenAPI specification that includes a suite of tools for auto-generating documentation, client SDK generation, and more. In contrast to the term OpenAPI, Swagger now refers to API tooling related to the OpenAPI spec. Some of these tools include Swagger Editor, Swagger UI, Swagger Codegen, SwaggerHub, and others. These tools are managed by Smartbear. Note: Although ‘Swagger’ was the original name of the OpenAPI spec, the name was later changed to OpenAPI to reinforce the open, non-proprietary nature of the standard. OpenAPI is still often referred to as Swagger.
- URI (Uniform Resource Identifier)
- A string of characters that identify a name or a resource. In RESTful APIs, URIs are used to identify resources.
API method terms
These terms are all related to API methods.
- CRUD
- Create, Read, Update, Delete. These four programming operations are often compared to POST, GET, PUT, and DELETE with REST API operations.
- DELETE
- An HTTP method that removes a resource.
- GET
- An HTTP method that retrieves a resource. GET requests do not modify any resources.
- HTTP method
- The type of action indicated in an API request, such as GET, POST, PUT, DELETE. Determines if you are reading, creating, updating, or deleting a resource. Synonymous with HTT verb and similar to HTTP operation.
- HTTP operation
- Refers to a combination of an HTTP method and a specific URI or endpoint. For example, a GET request to /users might retrieve a list of users, while a POST request to the same endpoint might create a new user. So, the combination of GET with /users and POST with /users indicates two distinct operations. Thus, an operation provides a more comprehensive view, as it takes into account both the action (HTTP method/verb) and the target (URI or resource).
- HTTP verb
- Same as HTTP method. The term ‘verb’ is used because each method/verb indicates an action or behavior.
- Idempotent methods
- HTTP methods where multiple identical requests should have the same effect as a single request. For instance, GET, PUT, and DELETE are idempotent, but POST is not.
- operation
- The type of API call, such as GET, POST, PUT, DELETE. Indicates allowed interactions with a resource.
- POST
- An HTTP method that creates a new resource.
- PUT
- An HTTP method that updates an existing resource or creates a new resource if it doesn’t exist.
API parameters terms
These terms are all related to API parameters.
- Accept header
- Part of the HTTP specification, it indicates the types of media that the client can process. For instance, specifying Accept: application/json will let the server know that the client expects JSON data.
- authentication token
- A token used to validate the identity of the client or user making the request. Synonymous with Authorization tokens or bearers.
- authorization tokens or bearers
- A type of header parameter, these are tokens used to identify and authenticate the user making the request. Commonly used with JWT (JSON Web Tokens) and OAuth.
- base URL
- The main part of the URL, which usually doesn’t change. Parameters are then added to this base URL to access different resources or perform specific operations. Similar to endpoint.
- Bearer token
- A type of access token that is passed in the header for API requests to authenticate the user. See authorization tokens or bearers.
- caching headers
- Headers like ETag, Last-Modified, Cache-Control, and Expires that help control how responses are cached by the client or intermediate proxies.
- headers
- Metadata sent with both API requests and responses. Headers can include information about the type of content being sent, authorization details, and more.
- header parameters
- Parameters that are included in the request header, usually related to authorization.
- OAuth
- An open-standard authorization protocol that allows third-party services to exchange your information without exposing your password.
- parameters
- Options that can be passed with an endpoint to influence the response, such as specifying the response format or number of results returned. Common types are header, path, and query string parameters.
- path parameters
- Parameters that appear within the path of the endpoint, before the query string (?). Path parameters are usually set off within curly braces {}.
- query string parameters
- The part of a URL after the ? symbol that contains parameter names and values to configure an endpoint request. Multiple parameters are separated with &.
- rate limit headers
- Headers that inform the client about how many requests they can make in a given timeframe and when they can make additional requests after reaching the limit.
- request body
- The data submitted in the body of the request, often used to create or update a resource. Defined in OpenAPI under requestBody. Synonymous with request payload.
- request example
- A sample API request showcasing how the endpoint should be accessed, including any required headers, parameters, or body content.
- request payload
- Same as request body.
- response headers
- Information, in key-value pairs, sent in the response from the server. They can provide metadata about the response data, indicate caching rules, or specify any cookies to be stored.
API responses terms
These terms are all related to API responses.
- Content-Type header
- Part of the HTTP specification, it’s used to indicate the type of data contained in the body of the message, whether it’s in an HTTP request or response. It allows the client or server to interpret how the data should be processed.
- error message
- A descriptive message accompanying error status codes to provide more context on the nature of the error.
- payload
- The data returned in the body of an API response. The payload contains the requested information or data from the API.
- response body
- The data returned by the server in response to a client’s request. It can contain information like resources (in case of a GET request) or status messages (like error messages). Usually in structured formats such as JSON or XML.
- response code
- HTTP status codes returned with API responses indicating success, failure, errors, etc.
- response example
- Shows a sample response from the request example. The response example is typically not comprehensive of all parameter configurations or operations, but it does correspond with the parameters passed in the request example.
- response headers
- Information, in key-value pairs, sent in the response from the server. They can provide metadata about the response data, indicate caching rules, or specify any cookies to be stored.
- response schema
- The description of the response from an API endpoint. The response schema documents the response in a more comprehensive, general way, listing each property that could possibly be returned, what each property contains, the data format of the values, the structure, optional/required aspects, and other details.
- status code
- A 3-digit number returned with an API response indicating whether the request succeeded or failed. Common status codes include 200 (success), 400 (bad request), 404 (not found), 500 (server error).
Documentation tools terms
These terms are all related to documentation formats and tools.
- API Blueprint
- The API Blueprint spec is an alternative specification to OpenAPI or RAML. API Blueprint is written in a Markdown-flavored syntax. See API Blueprint in this course, or go to API Blueprint’s homepage to learn more.
- Asciidoc
- A lightweight text format that provides more semantic features than Markdown. Used in some static site generators, such as Asciidoctor or Nanoc.
- continuous delivery
- Synonymous for continuous integration/continuous deployment (CI/CD).
- continuous integration/continuous deployment (CI/CD)
- Automation practices where changes to code are automatically tested and deployed. Useful for constantly updating documentation sites.
- DITA
- Darwin Information Typing Architecture, an XML-based architecture for authoring, producing, and delivering technical information. Allows topic-based authoring.
- docs-as-code
- Treating documentation files like code by using lightweight markup, version control, plain text editors, and engineering tools/workflows. Facilitates developer contributions.
- documentation pipeline
- The automated process that transforms raw source content, often in Markdown or other formats, into a live documentation site.
- documentation theme
- A predefined set of styles, layouts, and behaviors applied to documentation generated by static site generators or documentation tools.
- frontmatter
- Metadata at the beginning of a Markdown or other lightweight markup language file. Typically written in YAML and defines site-specific attributes.
- HAT
- Help Authoring Tool. Refers to the traditional help authoring tools (RoboHelp, Flare, Author-it, etc.) used by technical writers for documentation. Tooling for API docs tends to use docs-as-code tools more than HATs.
- Hugo
- A static site generator that uses the Go programming language as its base. Along with Jekyll, Hugo is among the top 5 most popular static site generators. Extremely fast site generation time.
- i18n
- Short for internationalization. There are 18 letters between i and n in the word internationalization. See localization/internationalization.
- Java
- General purpose programming language commonly used in enterprise application development. Preferred by some organizations over languages like PHP.
- Javadoc
- A documentation generator that produces API reference documentation from Java source code comments formatted with tags. The Javadoc tool is the standard for documenting Java APIs.
- JSON
- JavaScript Object Notation. A common data format for API responses, consisting of attribute-value pairs and arrays. OpenAPI (Swagger) specifications are often written in JSON. YAML tends to be more human-readable, while JSON is often easier for machines to process.
- l10n
- Short for localization. There are 10 letters between the l and n in localization. See Localization/Internationalization.
- Localization/Internationalization (l10n/i18n)
- The process of adapting documentation to different languages and regions.
- Markdown
- A lightweight markup language that uses plain text formatting syntax that gets converted to HTML. Popular format with developers writing documentation.
- markup language
- A system for annotating content to represent its structure and presentation. Examples include Markdown and XML.
- metadata
- Data about data. In documentation, this refers to information about the document itself added to the file, like authors, dates, tags.
- open source
- Software projects with publicly available source code that can be used, modified, and distributed by anyone. Provide opportunities to contribute.
- OxygenXML
- An XML editor and publishing platform that supports DITA and other XML formats, along with Markdown and docs-as-code workflows.
- RAML
- Stands for REST API Modeling Language and is similar to OpenAPI specifications. RAML is backed by Mulesoft, a commercial API company, and uses a more YAML-based syntax in the specification.
- ReadTheDocs
- A platform that automatically builds and deploys documentation from repositories, especially popular for open source projects.
- reStructuredText (reST)
- A lightweight markup language often used with Sphinx. Offers more directive capabilities than Markdown.
- schematron
- XML vocabulary that allows validating the structure and content in XML documents against a set of rules. Can help enforce standards.
- Sphinx
- A static site generator developed for managing documentation for Python. Sphinx is a documentation-oriented static site generator available that includes features such as search, sidebar navigation, semantic markup, and managed links. Based on Python.
- static site generator
- Tool that compiles a website from simpler text-based source files like Markdown. Popular static site generators include Jekyll, Hugo, and Sphinx. A common part of docs-as-code workflows.
- taxonomy
- The classification and organization of documentation content, often using tags, categories, or other metadata.
- templating language
- Languages like Liquid, Handlebars, or Go that allow inserting dynamic content into static templates when sites are built. Help abstract complex site generation logic.
- versioning (documentation)
- The practice of keeping multiple versions of documentation to align with different versions of the product or software. See also API versioning.
- YAML
- A human-readable data serialization format commonly used for configuration files and OpenAPI definitions. Uses indentation and whitespace for structure. Less visually noisy than JSON. Recursive acronym for YAML Ain’t No Markup Language.
Version control terms
These terms are all related to version control.
- blame
- A Git command used to display who made the last modification to each line of a file and what that modification was.
- branch
- A copy of the Git repository that is often used for developing new features. Usually, you work in branches and then merge the branch into the master branch when you’re ready to publish.
- Bitbucket
- Similar to GitHub and GitLab, Bitbucket is a platform (by Atlassian) that hosts Git repositories and facilitates collaboration, version control, and often continuous integration/continuous deployment (CI/CD).
- cherry-pick
- Allows you to select a specific commit from one branch and apply it onto another branch.
- clone
- The Git command used to copy a repository in a way that keeps it linked to the original. The first step in working with any repository is to clone the repo locally. Git is a distributed version control system, so everyone working in it has a local copy (clone) on their machines.
- commit
- A snapshot of your changes to the Git repo. Git saves the commit as a snapshot in time that you can revert to later if needed. You commit your changes before pulling from origin or before merging your branch within another branch.
- checkout
- The process of retrieving a specific revision or branch from a repository to work on locally.
- fork
- A personal copy of another user’s repository. This allows one to experiment with changes without affecting the original project.
- Git
- A distributed version control system that tracks changes to code or text files. Allows branching and merging of file updates among multiple collaborators. Core part of collaborating on software projects and docs-as-code workflows.
- Git hook
- Scripts that can run automatically on occurrence of specific events in a Git repository. They can be useful for automating certain tasks like code checks.
- GitHub
- A web-based platform built around Git that provides user interfaces and collaboration features such as wikis, issue tracking, and pull requests. Commonly used to manage documentation.
- GitLab
- Similar to GitHub and Bitbucket, GitLab is a platform that hosts Git repositories and facilitates collaboration, version control, and often continuous integration/continuous deployment (CI/CD).
- Git repo
- In Git, a repo (short for repository) stores your project’s code. Usually, you only store non-binary (human-readable) text files in a repo, because Git can run diffs on text files and show you what has changed.
- HEAD
- A pointer/reference to the latest commit in the branch you’re currently on.
- Main branch
- The primary branch where all the development is done and from where new branches are created. Previously referred to as the master branch but changed for inclusivity reasons.
- merge conflict
- Occurs when two branches have changes in the same part of a file, and Git cannot automatically determine which version to use.
- Mercurial
- A distributed revision control system, similar to Git but not as popular.
- Perforce
- Revision control system often used before Git became popular. Often configured as a centralized repository instead of a distributed repository.
- pull
- In Git, when you pull from origin (the main location where you cloned the repo), you get the latest updates from origin onto your local system.
- pull request
- GitHub workflow where proposed code changes in a branch are submitted for review and potential merging into the project’s main branch.
- push
- In Git, when you want to update the origin (the main location where you cloned the repo) with the latest updates from your local copy, you run git push. Your updates will bring origin back into sync with your local copy.
- rebase
- A way to integrate changes from one branch into another. It’s an alternative to merging and involves reapplying changes from one line of work onto another in a sequential manner.
- remote
- A reference to an external repository, typically hosted on a server, where teams collaborate on a project. It allows developers to fetch data from or push data to the external repository. The default remote is usually named ‘origin’ when you clone a repository.
- stash
- A temporary space to store changes that you don’t want to commit yet. This allows you to switch branches without committing your current changes.
- Subversion (SVN)
- Centralized version control system developed by Apache. It was one of the most popular version control systems before the rise of Git.
- tag (Git)
- A reference to a specific commit. Tags are typically used to capture a point in history, often used for release versions (e.g., v1.0).
- version control
- A system to track changes to code and documentation over time, support collaboration, and maintain previous versions. Examples are Git, SVN, Perforce. Also referred to as version control system.
- webhooks
- Automated messages sent when a specific event happens on a platform. In docs-as-code, often used to trigger builds or updates when changes are pushed to a repository.
Writing process terms
These terms are all related to the writing process.
- cross-reference
- A link from one documentation topic to a related topic to help users navigate and find associated information.
- outline
- A list of section headings and bullet points that maps out the structure and content to be covered in a document. Outlines are created before writing begins.
- peer review
- The process where fellow technical writers or other professionals review the documentation for clarity, consistency, and accuracy.
- portfolio
- A collection of writing samples and projects that demonstrates a technical writer’s skills and experience. Useful for job applications.
- publishing
- The act of finalizing documentation and making it publicly available on a website or elsewhere. The last step of the writing process.
- review
- The process of having stakeholders and subject matter experts read draft documentation and provide feedback to improve the content.
- stakeholder
- People involved in some way with a product, such as engineers, product managers, executives, partners, support, and others. Stakeholders review documentation.
OpenAPI terms
These terms are all related to OpenAPI.
- $ref (OpenAPI spec)
- Allows the inclusion of a piece of an OpenAPI description from another location, aiding in reusability.
- API Console
- Renders an interactive display for the RAML spec. Similar to Swagger UI, but for RAML. See also interactive API console.
- components (OpenAPI spec)
- Storage for re-usable schema definitions referenced elsewhere through $ref pointers in the OpenAPI specification.
- externalDocs (OpenAPI spec)
- Links to external documentation, providing more in-depth information or context about the API or specific endpoints.
- info (OpenAPI spec)
- Provides metadata about the API like title, description, version, and other details.
- mock server
- A simulated API server that returns sample responses without any actual implementation or processing of requests. Mock servers allow testing interactions with an API before it’s built.
- OAS
- Abbreviation for OpenAPI specification.
- OpenAPI contract
- Synonym for OpenAPI specification document.
- OpenAPI Initiative
- The governing body that directs the OpenAPI specification. Backed by the Linux Foundation.
- OpenAPI specification
- A vendor-neutral specification (in JSON or YAML) for describing REST APIs. Allows API providers to describe API operations, parameters, authentication methods, models, and other components in a portable document. When valid, the specification document can be used to create interactive documentation, generate client SDKs, run unit tests, and more. See also Swagger.
- OpenAPI specification document
- The file (either in YAML or JSON syntax) that describes your REST API. Follows the OpenAPI specification format. See also API contract
- OpenAPI (Swagger)
- A specification for describing REST APIs that can be used to generate interactive documentation, code libraries, and more.
- parameters (OpenAPI spec)
- In the OpenAPI spec, ‘parameters’ specifies the expected inputs for API operations, such as path, query, and header parameters.
- paths (OpenAPI spec)
- In the OpenAPI spec, ‘paths’ denote the available routes or endpoints in an OpenAPI specification.
- responses (OpenAPI spec)
- In the OpenAPI spec, ‘responses’ describes the expected responses from API operations.
- securitySchemes (OpenAPI spec)
- Defines the authentication methods used by the API, like API keys, HTTP authentication, OAuth, etc.
- servers (OpenAPI spec)
- Defines the base URLs for the API’s endpoints, allowing the specification to describe APIs with varying environments like development, staging, or production.
- tags (OpenAPI spec)
- Groups for endpoints to organize them in the interactive documentation. Used in the OpenAPI spec.
- validation
- The process of ensuring that an OpenAPI document adheres to the OAS specification’s structure and constraints.
Swagger terms
These terms are all related to Swagger.
- API definition
- See API specification. This is the structured description of how the API functions, its endpoints, parameters, responses, and more. Swagger tools parse this definition to provide various functionalities.
- Interactive API console
- Provided by Swagger UI, this console allows users to make API calls directly from the documentation. This interactive feature enables developers to try out the API endpoints as they read through the docs.
- Swagger
- A framework for the OpenAPI specification that includes a suite of tools for auto-generating documentation, client SDK generation, and more. In contrast to the term OpenAPI, Swagger now refers to API tooling related to the OpenAPI spec. Some of these tools include Swagger Editor, Swagger UI, Swagger Codegen, SwaggerHub, and others. These tools are managed by Smartbear. Note: Although ‘Swagger’ was the original name of the OpenAPI spec, the name was later changed to OpenAPI to reinforce the open, non-proprietary nature of the standard. OpenAPI is still often referred to as Swagger.
- Swagger annotations
- In-code annotations that developers can use within their codebase. When the application code is processed by Swagger tools, these annotations help auto-generate an OpenAPI specification.
- Swagger Codegen
- Generates client SDK code for a lot of different platforms (such as Java, JavaScript, Scala, Python, PHP, Ruby, Scala, and more). The client SDK code helps developers integrate your API on a specific platform and provides for more robust implementations that might include more scaling, threading, and other necessary code. In general, SDKs are toolkits for implementing the requests made with an API. Swagger Codegen generates the client SDKs in nearly every programming language.
- Swagger Editor
- An online editor that validates your OpenAPI document against the rules of the OpenAPI specification. The Swagger Editor will flag errors and give you formatting tips.
- SwaggerHub
- A premium API platform from Smartbear that provides collaboration, mocking, testing, and publishing tools around the OpenAPI specification.
- Swagger Inspector
- An online tool for testing APIs. It helps you quickly validate and inspect API requests and responses without the need for any underlying implementation.
- Swagger Petstore
- A basic example of an OpenAPI specification rendered by Swagger. Many people use this as a template or a reference when creating their own API specifications.
- Swagger plugins
- Extensions or plugins available for various frameworks and platforms to integrate Swagger capabilities into the software development workflow. Examples include Maven plugins, Gradle plugins, etc.
- Swagger UI
- An open-source web framework (on GitHub) that parses an OpenAPI specification document and generates an interactive documentation website. Swagger UI is the tool that transforms your spec into the Petstore-like site.
Project management terms
These terms are all related to project management.
- acceptance criteria
- Specific conditions that a user story or feature must satisfy to be accepted by the product owner or stakeholders.
- backlog
- In Scrum, a prioritized list of work items or user stories waiting to be worked on and completed by the team.
- Backlog grooming/refinement
- An ongoing process where the product owner and the Scrum team review items in the backlog to ensure they are prioritized and ready for future sprints.
- daily standup
- A short daily meeting, usually held in the morning, where each member of the Scrum team describes what they accomplished yesterday, plans for today, and any blocking issues.
- definition of done
- In Scrum, the agreed upon criteria that user stories must meet before they can be considered complete and shippable.
- epic
- A large user story that can be broken down into smaller stories, used in Agile software development to group related functionality.
- iteration
- Synonymous with sprint.
- Kanban
- An agile project management methodology that uses a board with columns for tracking the progress of work through different states.
- product owner
- In Scrum, the person responsible for maintaining the product backlog, setting priorities, and ensuring the team delivers maximum value to the stakeholders.
- release planning
- A longer-term planning meeting where the team determines which features or user stories are targeted for an upcoming release.
- Scrum
- An agile project management framework that breaks down product development into short, iterative sprints with daily standup meetings.
- sprint
- In Scrum, a short, timeboxed period (usually 1-4 weeks) focused on completing specific work items for a product increment.
- sprint demo
- A meeting at the end of each sprint where the Scrum team shows completed user stories/features to stakeholders and collects feedback.
- sprint planning
- A meeting at the start of each sprint where the product owner and team select user stories from the backlog to work on that align with the sprint goal.
- sprint retrospective
- A meeting at the end of each sprint where the Scrum team reflects on what went well, what needs improvement, and lessons learned from the sprint.
- stakeholder engagement
- The process of involving all parties that have an interest in the project, ensuring their feedback and concerns are considered.
- story points
- A unit of measure for expressing the overall size, complexity, and effort required to implement a user story or feature in Agile methodologies like Scrum.
- timeboxing
- Allocating a fixed amount of time for an activity. Used in Scrum for meetings and sprints to ensure they don’t overrun.
- T-shaped skills
- The concept that members of the team should have deep expertise in a specific discipline (the vertical line of the T) and broad skills and knowledge across many disciplines (the horizontal line of the T). This encourages collaboration and flexibility within teams.
- user story
- A high-level definition of a requirement or feature from an end-user perspective, used as the basis for planning in Agile methodologies.
- velocity
- In Scrum, the amount of work a team can successfully complete within a sprint, measured in story points. Used to forecast how much work can be done.
- waterfall
- A sequential, linear software development methodology where phases must be completed in order before moving to the next phase.
- Work in Progress (WIP)
- In Kanban, a limit set to restrict the number of items being worked on simultaneously. It ensures that teams are not overwhelmed and can maintain focus.
Testing and QA terms
These terms are all related to testing and quality assurance.
- accessibility testing
- Ensuring that documentation is usable and understandable by people with disabilities, in compliance with standards like the Web Content Accessibility Guidelines (WCAG).
- agile testing
- Relying on user feedback after release to improve docs iteratively. Risky without pre-release testing.
- assumption
- An idea taken for granted without confirmation. Docs often make faulty assumptions about user knowledge.
- beta testing
- Testing done by real users in their real environment before the final release. Feedback from beta testing can highlight issues in both the product and the associated documentation.
- bug
- A defect or issue in the product or documentation. Bugs raised in documentation might pertain to factual inaccuracies, unclear instructions, or missing content.
- feedback loop
- A process for continuously gathering and integrating feedback into documentation, typically using comments, surveys, or direct user input.
- localization testing
- Ensuring that the translated versions of documentation are accurate, culturally appropriate, and align with the original intent of the content.
- QA
- Quality Assurance. Team responsible for testing products before release to ensure quality.
- regression testing
- Testing conducted to ensure that recent changes or updates haven’t negatively impacted the existing documentation or functionality.
- smoke test
- Preliminary testing to catch the most glaring and obvious errors in documentation, such as broken links or missing pages.
- test case
- A scenario to execute to validate expected behavior and responses. QA teams run test cases.
- test environment
- The system setup used to experiment with and validate code, APIs, apps, etc. Often a separate test server.
- usability testing
- A method to evaluate the effectiveness and clarity of documentation by observing real users as they try to achieve tasks using the documentation.
- user testing
- Having real users try documentation to identify problems and gaps. Essential for creating usable docs.
Conceptual docs terms
These terms are all related to conceptual documentation.
- authorization documentation
- Instructions and details on how users can obtain access credentials and authenticate their requests to the system. Crucial for APIs that require secure access.
- code sample
- Practical, executable examples in various programming languages that show how to use specific features or accomplish tasks with the product.
- conceptual docs
- Explains foundational ideas, giving readers a sense of the bigger picture. Guides users in understanding the underlying principles and concepts of the technology or product.
- error message documentation
- Explanations for potential errors users might encounter, offering solutions or workarounds to address these issues.
- getting started tutorial
- A beginner’s guide aimed at helping users achieve their first success with the product or technology. Simplifies the initial experience and encourages deeper exploration.
- product overview documentation
- Introductory content that offers a bird’s-eye view of the product, its main features, and benefits. It sets the stage for users, helping them understand what the product is and why it matters.
- quick reference
- A condensed sheet or guide that highlights the most important elements, often used for quick consultations or reminders.
- rate limiting and thresholds documentation
- Guidelines on the number of requests users can make within a specified time frame, ensuring the system remains responsive and available to all users.
- reference docs
- Detailed documentation of an API’s resources/endpoints, parameters, sample requests, responses, errors, etc. Provides a reference for developers.
- release notes
- Updates accompanying each new version of the product, detailing the additions, changes, bug fixes, and any other relevant information.
- support
- Resources that help API users, often provided through live chat, forums, or ticketing systems.
- terminology
- Specialized words and phrases used in a field. See glossary.
- tutorial
- Detailed guides focused on teaching users how to achieve specific tasks. Broken down step-by-step, often accompanied by screenshots, code snippets, or videos.
API tools and platform terms
These terms are all related to API tools and platforms.
- API Transformer
- A cross-platform service provided by APIMATIC that will automatically convert your specification document from one format or version to another. See apimatic.io/transformer.
- Apiary
- Platform that supports the full life-cycle of API design, development, and deployment. For interactive documentation, Apiary supports the API Blueprint specification, which is similar to OpenAPI or RAML but includes more Markdown elements. It also supports the OpenAPI specification now too. See apiary.io.
- Apigee
- Similar to Apiary, Apigee provides services for you to manage the whole lifecycle of your API. Specifically, Apigee lets you ‘manage API complexity and risk in a multi- and hybrid-cloud world by ensuring security, visibility, and performance across the entire API landscape.’ Supports the OpenAPI spec. See apigee.com.
- APIMATIC
- Supports most REST API description formats (OpenAPI, RAML, API Blueprint, etc.) and provides SDK code generation, conversions from one spec format to another, and many more services. For example, you can automatically convert Swagger 2.0 to 3.0 using the API Transformer service on this site.
- Blobr
- A cloud platform for creating API portals that package API use cases into purchasable products with customized documentation. Allows monetizing APIs.
- customization
- Changing the default styling and branding of a platform. Blobr enables customizing the portal name, logo, colors, domain, etc.
- Mulesoft
- Similar to Apiary or Apigee, Mulesoft provides an end-to-end platform for designing, developing, and distributing your APIs.
- monetization
- Generating revenue from an API, through methods like usage fees, rate limits, subscriptions, or freemium models.
- Postman
- A GUI application for interacting with APIs by constructing requests and viewing responses. Simplifies API testing. Useful for exploring and trying out APIs.
- RAML Console
- In Mulesoft, the RAML Console is where you design your RAML spec. Similar to the Swagger Editor for the OpenAPI spec.
- Redoc
- An open-source tool for generating interactive API reference documentation from OpenAPI (formerly Swagger) definitions. Provides an expandable three-column layout.
- Redocly
- Company that offers premium tools and services for API documentation, including enhanced Redoc, CLI tools, and developer portals.
- Repo
- A tool for consolidating and managing many smaller repos with one system.
- Smartbear
- The company that maintains and develops the Swagger tooling, such as Swagger Editor, Swagger UI, Swagger Codegen, SwaggerHub, and others.
- Stoplight
- Provides a platform with visual modeling tools to create an OpenAPI document for your API — without requiring you to know the OpenAPI spec details or code the spec line by line.
About Tom Johnson
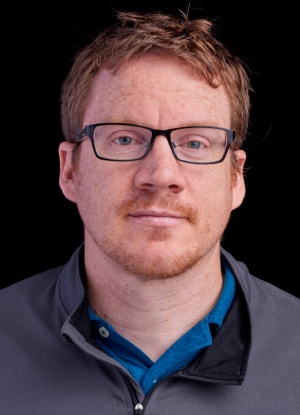
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.
151/165 pages complete. Only 14 more pages to go.