JavaScript: Strings
Strings are non-numbers assigned to variables. You surround strings in quotes, like this:
var endMessage = "Congratulations! You're finished.";
If you have a quote inside a quote, you can add a back slash to tell JavaScript to ignore the quotation mark (this is called "escaping"). Otherwise, the quotation mark inside the string would end your string. In the following example, the quotations around relax
are escaped.
var endMessage = "Congratulations. Take a break and
find some time to \"relax\" a bit.";
Single quotes inside double quotes are allowed without the need to escape them. If you have contractions or possessives, which use single quote marks, make sure you surround the string with double quotes.
var endMessage = "Congratulations, it's all yours.";
Strings as Arrays
Variables with strings can be treated as arrays. If you add .length
to the variable, JavaScript returns the number of characters in the string.
var endMessage = "Congratulations! You're finished.";
//write the character length of the string.
console.log(endMessage.length);
The result is 33.
Changing Strings to Upper or Lower Case
You can also convert the string to upper case with the .toUpperCase
method:
var endMessage = "Congratulations! You're finished.";
//write the string to upper case
console.log (endMessage.toUpperCase() );
The result is CONGRATULATIONS! YOU'RE FINISHED.
Similarly, you can use .toLowerCase
to convert the string to lowercase.
Splitting Apart Strings
You can split the string apart into different positions. To do this, you add use the .split
method.
var endMessage = "Congratulations! You're finished.";
//split the string at the spaces
console.log (endMessage.split(" ");
This splits the string up into an array, with each word assigned to a position. Congratulations!
is at position 0. You're
is at position 1. And finished.
is at position 2.
The empty space in parentheses (" ")
means to split the string apart at the empty space. You could also split the string apart at a comma or some other mark. To split the string apart by commas, you add (",")
instead of the empty space.
Finding the Position for a Word in the String
You can find the exact position for a word in a string. To do this, use the .indexOf
method:
var endMessage = "Congratulations! You're finished.";
//find the position of the word "finished"
console.log ( endMessage.indexOf("finished.") );
The word finished
appears in position 2. (Remember that 0 is the starting point, not 1.)
You can use .lastIndexOf
to find where the last instance of a word appears.
var endMessage = "Congratulations, Jon! Now go home, Jon.";
//find the last instance where Jon appears
console.log( endMessage.lastIndexOf("Jon") );
The result is 49. The last instance of "Jon" appears 49 characters from the start.
If the word can't be located, JavaScript returns a -1
. This -1
result can be useful for conditional statements. For example, you can ask whether a certain word is found. If not, then you can take a specific action.
For a full reference of JavaScript commands, see the Mozilla JavaScript reference.
About Tom Johnson
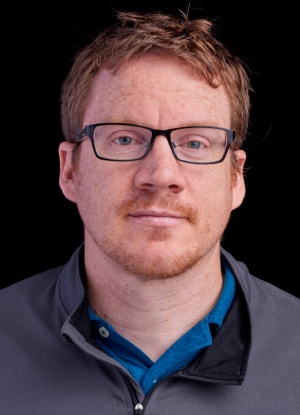
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.