JavaScript: Arrays
Arrays are variables that have multiple values. You normally write out variables like this:
var game = 49;
However, an array stores multiple values for the same variable. It sets each value at different index positions. A sample array might be as follows:
var game[0] = 49;
var game[1] = 56;
var game[2] = 63;
var game[3] = "Touchdown!"
var game[4] = 70;
The positions for each index in the array are listed in brackets after the variable. Arrays always start at an index of 0 (not 1) and increment upward.
Listing out each position in the array on its own line is lengthy, so there's a shorter way to list the positions in the array:
var game = [ 49,56,63,"Touchdown!",70 ];
The order of the values reflects their positions. 49
corresponds to position 0, 56
corresponds to position 1, 63
corresponds to position 2, and so on.
If you want to get the value from an array, add the position you want in brackets after the array's name:
game[4];
This would return the value Touchdown!
.
Alternative Ways of Writing Arrays
Although brackets are common ways of writing arrays, you can also write arrays like this:
var game = Array();
or like this:
var game = new Array();
You would then include the positions of the array in the parentheses:
var game = new Array(49,56,63,"Touchdown!", 70);
Methods for Arrays
Arrays are considered objects, and as objects, you can apply methods to them. Methods are the ways you can manipulate objects. Think of methods as various functions that you can apply to an object.
For example, if you want to see how many positions you have in your array, add .length
to the array name:
//declare an array
var game = [ 49,56,63,"Touchdown!",70 ];
//write the number of positions the array has
console.log(game.length);
The result would be 5.
Methods are always added to the object name after a dot.
Some other methods include:
.reverse //lists the array in reverse order
.join //joins the values of the array
.sort //sorts the values of the array
About Tom Johnson
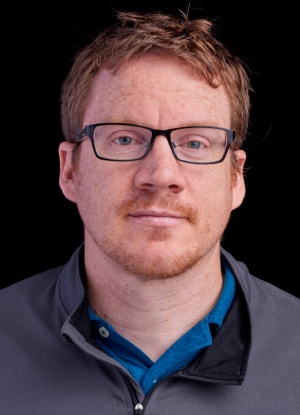
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.