JavaScript: Conditional Code
You can start building more complex JavaScript statements by using conditional code. The conditional code allows you to ask if a condition is met. If the condition is met, a certain code block is run. If the condition isn't met, a different code block is run. The answer to the condition must always be a yes or no answer.
Conditional Code Format
Here's the format of the conditional code:
//begin with an if statement
if ( condition ) {
//insert code to run ...
alert("Hello World");
//otherwise, run this code
} else {
//end the code block
}
The statement begins with if
followed by a condition in parentheses. After the condition, the code block is run. Code blocks are contained within curly braces { }
. The else
means "if the previous condition isn't met, do this instead," or more simply, "Otherwise."
Here's an example with some information:
//first declare a variable
var a = 100;
//state an if condition
if ( a > 100 ) {
//if true, then run this command
alert("Hello");
//if the condition isn't met, do this:
} else {
alert("Goodbye");
}
Conditional Operators
In the condition statement, you can use a variety of operators to ask questions:
if ( a == b) // if a is equal to b
if ( a !=b ) // if a is not equal to b
if ( a > b ) // if a is greater than b
if ( a < b ) // if a is less than b
if ( a >= b ) // if a is greater than or equal to b
if ( a <= b )// if a is less than or equal to b
Equals Signs
Note that the equals operator is two equals signs ==
, not one. When you set the value of a variable, you use one equals sign =
. One equals sign means "assign as" whereas two equals signs means "equals".
There's also a triple equals sign ===
, which means "is strictly equal to." This is a more formal way of checking conditions. For example, if you have a variable that's a number, such as var a = 10
, and another variable that's a string, such as var a = "10"
, the two variables are different types of variables and therefore aren't "strictly" equal, even though they're the same.
Nested Conditions
You can nest multiple conditions. A nested condition looks like this:
if ( condition ) {
//run some code...
} else {
if ( condition ) {
//run some code...
} else {
// otherwise, run this code
}
Usually you don't nest more than a couple of condition levels, so that the code doesn't become too complicated.
And/Or Operators
You can create more advanced conditions by including &&
(and) or ||
(or) operators. Here's an example of the "and" operator:
//if a is equal to b and a is equal to c
if ( a == b && a == c )
Here's an example with "or" operator:
//if a is equal to be or a is equal to c
if ( a == b || a == c )
For clarity, you can surround the conditions in their own parentheses, like this:
if ( (a == b) && (a == c) )
Just remember that you always need both opening and closing parentheses. And just as with more simple conditional statements, the answer needs to be either yes or no.
Arithmetic Operators
You can use a variety of arithmetic operators:
+ // add
- //subtract
* //multiple
/ //divide
% // the remainder (the % is called a modulus)
Here's an example:
if ( a + b == 100)
This is pretty straightforward: if a plus b is equal to 100. However, when you combine multiple arithmetic operators, JavaScript runs multiplication and division first, and then addition and subtraction. This prioritization is called "operator precedence." So if the operation is the following:
( 2 + 2 * 12 )
The result will be 26 rather than 48. You multiply 12 * 2 to get 24, and then add 2 to get 26.
To specify that the addition or subtraction runs first, you can surround it in parentheses:
( (2+2) * 12 )
Now the result will be 48.
The Remainder Operator
The remainder operator refers to what's left over when a number doesn't divide evenly into another number. Here's an example of the remainder operator in action:
//set a variable
var year = 2010;
//set a variable to be the days left over after
//dividing the year by 4
var excess = year % 4
The value for the remainder would be 2. This is because 4 goes into 2010 (the year) 502 times, with a remainder of 2.
Arithmetic Shorthand
Because arithmetic operators are used so frequently, there's a shorthand way of writing them. When you see +=
, it means to add the number that follows to the variable. Conversely, -=
means subtract the number from the variable, and so on.
The following are the long and short ways of writing the same operators:
score = score + 10; // the long way
score += 10; // the short way</p>
<p>score = score - 10; // the long way
score -= 10; // the short way</p>
<p>score = score * 10; // the long way
score *= 10; // the short way</p>
<p>score = score / 10; // the long way
score /= 10; // the short way
Unary (++
) Operators
There's another shorthand for arithmetic operators: ++
is called a unary operator. When you add ++
with a variable, it means to increment the variable by 1. Similarly, if you add --
, it means decrease the variable by 1. Here are a few examples:
a = a + 1; // the long way of writing it
a += 1; // a shorter way
a ++; // an even shorter way
a++; // even shorter
++a; // also acceptable and means the same thing.
Ternary Operators
There's one more shorthand operator to mention. A ternary operator has three components and reduces what would otherwise occupy several lines of code into just one line. Rather than writing out:
//declare some variables
var a = 500;
var b = 600;
var highScore;
//state an if condition
if ( a > b ) {
//run some code
highScore = a;
//otherwise run this code
} else {
highScore = b;
}
You can compress this into a couple of lines in the following format:
condition ? true : false
After stating the condition, add a question mark ?
. After the question mark, write what should happen if the condition is true; then add a colon. On the right of the colon, write what should happen if the condition is false.
Here's how the ternary operator would be written using the previous example:
var a = 500; b = 600;
var highScore = ( a > b ) ? highScore = a : b;
In other words, if a
is greater than b
, assign the highScore
variable as a
. If it's not, assign the highScore
variable as b
.
About Tom Johnson
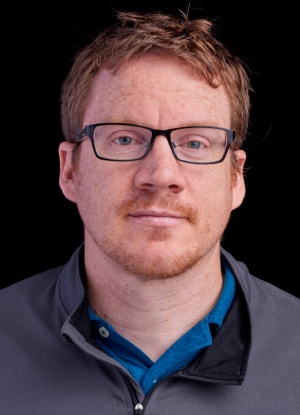
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.