Java: Casting
Quick summary
- A way of converting one value type to another
Eclipse example: casting
Detailed description
Casting is a way of converting one value type to another. In Java, values have value types allotted for them. You don’t just create a variable and set it to any number, because the system has to reserve space for that value. Larger values need larger amounts of space reserved.
byte 2;
int 600;
It would be inefficient to allot all values the same space in your system. You can change the value types from one to another. This is called casting.
Suppose you have this:
byte myByte = 20; // max is 27
short myShort = 67;
int myInt = 899;
long myLong = 88;
float myFloat = 235.5f;
double myDouble = 8000.7;
Now you want to convert your long to a double. You can’t do it because the double doesn’t fit into the long.
myLong = myDouble;
System.out.println(myLong);
However, the reverse would work:
myDouble = myLong;
System.out.println(myDouble);
But now you have the problem that you’re using more memory than needed to accommodate the myDouble. It only needs to hold a long value. So you cast it like this:
myDouble = (double)myLong;
System.out.println(myDouble);
This changes the value type to double.
More reading
About Tom Johnson
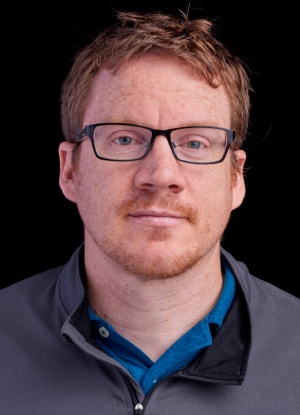
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.