Java: Setters
Quick Summary
- setters refers to a technique for encapsulating a value so that users don’t set invalid data types or other incorrect data as a field value.
Eclipse example: getter_and_setter_methods
Classes have instance variables, but usually users don’t need to know which variables are available in the class. Instead, it’s more common to provide methods that allow users to set the value of the instance variables.
In other words, rather than doing something like this to set the value:
myObject.name = "Tom";
It’s more common to use a setter method as follows:
package setters;
public class SleepTime {
int timev;
int time;
public int setSleepTime(int timev) {
int time = timev;
return time;
}
public int getSleepTime() {
return time;
}
}
<div class="container comicBlock">
<div class="row inpostComics">
<script>
var contents=new Array()
//Paligo
contents[0]='<div class="border col-md-5"><a class="noCrossRef" ref="nofollow" href="https://idbwrtng.com/paligoinline_apr24_2022"><img style="border: 1px solid darkgray" src="https://s3.us-west-1.wasabisys.com/idbwmedia.com/images/paligo_april.png" alt="Paligo" /></a><div class="comicLink"> <a href="https://idbwrtng.com/paligoinline_apr2_2023">Find out why Paligo is the choice for some of the world\'s leading brands.</a></div></div>'
//Xpublisher
contents[1]='<div class="border col-md-5"><a class="noCrossRef" ref="nofollow" href="https://idbwrtng.com/xpublisher-july17"><img style="border: 1px solid darkgray" src="https://s3.us-west-1.wasabisys.com/idbwmedia.com/images/xpublisher-idratherbewriting.jpg" alt="Xpublisher" /></a><div class="comicLink"> <a href="https://idbwrtng.com/xpublisher-july17">Explore Xpublisher, the workflow-based CMS for technical communication</a></div></div>'
//TWi
contents[2]='<div class="border col-md-5"><a class="noCrossRef" ref="nofollow" href="https://idbwrtng.com/twiinline_apr24_2022"><img style="border: 1px solid lightgray" src="https://s3.us-west-1.wasabisys.com/idbwmedia.com/images/technically_write_it.jpg" alt="TWi: Leading Provider of Documentation and Technical Communication Solutions" /></a> <div class="comicLink"><a href="https://idbwrtng.com/twiinline_apr24_2022">Which one are you, which one should you be, and why?</a></div></div>'
//Xeditor
contents[3]='<div class="border col-md-5"><a class="noCrossRef" ref="nofollow" href="https://idbwrtng.com/xeditor-july17"><img style="border: 1px solid darkgray" src="https://s3.us-west-1.wasabisys.com/idbwmedia.com/images/xeditor-idratherbewriting.jpg" alt="Xpublisher" /></a><div class="comicLink"> <a href="https://idbwrtng.com/xeditor-july17">Explore Xeditor, a customizable, web-based XML editor for any CMS</a></div></div>'
// Zoomin
contents[4]='<div class="border col-md-5"><a class="noCrossRef" ref="nofollow" href="https://idbwrtng.com/zoomin-publish-faster"><img style="border: 1px solid darkgray" src="https://s3.us-west-1.wasabisys.com/idbwmedia.com/images/zoominsquare.jpeg" alt="Zoomin" /></a><div class="comicLink"> <a href="https://idbwrtng.com/zoomin-publish-faster">Consolidate your content to improve self-service</a></div></div>'
var i=0
//variable used to contain controlled random number
var random
//while all of array elements haven't been cycled thru
while (i<contents.length){
//generate random num between 0 and arraylength-1
random=Math.floor(Math.random()*contents.length)
//if element hasn't been marked as "selected"
if (contents[random]!="selected"){
document.write(contents[random])
//mark element as selected
contents[random]="selected"
i++
}
}
</script>
</div>
</div>
<div style="clear:both;"></div>
and to set and retrieve them as follows:
package setters;
public class App {
public static void main(String[] args) {
SleepTime tomSleepTime = new SleepTime();
System.out.println(tomSleepTime.setSleepTime(11));
System.out.println(tomSleepTime.getSleepTime());
}
}
There’s a shortcut in Eclipse that makes it very easy to add getters and setters. Right click your class and select Source > Generate Getters and Setters. Here’s an example of what it produces:
public class Robot {
private int id;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
Here I had just entered private int id
. It automatically set the get and set methods for this value. It’s pretty common to set up getters and setters for field values of a class.
Note that the setter method uses this.id = id;
. That’s because you’ll create an object from the Robot class like this:
Robot myRobot = new Robot();
You would then set the value for the id like this:
myRobot.id = "some value";
To make it generic, you use this
instead. The word this
refers to whatever the object in scope is.
Encapsulation
Encapsulation means that an object holds its contents in such a way that other objects can’t see or change those contents (though we have a number of ways to provide access to the contents of a class). Java for Absolute Beginners
About Tom Johnson
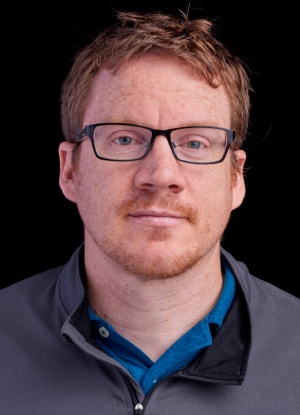
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.