Java: Getters and setters
Quick summary
- best practice technique for allowing users to set field values
- set method has user pass an argument;
this
keyword sets the argument as the value - get method retrieves the value of the argument set by the set method
- this technique helps protect code from users setting invalid values
Eclipse example: getter_and_setter_methods
When you create an instance variable in a class, highlight the variable, right-click, and select Source > Generate Getter and Setter methods. This will insert something like the following:
public class Keyboard {
private String functionKeys;
public String getFunctionKeys() {
return functionKeys;
}
public void setFunctionKeys(String functionKeys) {
this.functionKeys = functionKeys;
}
}
This allows you to easily change the String functionKeys as you need it. You’ve encapsulated it from users changing it, screwing it up, etc.
Now the user can set the value of functionKeys
through the setter method, and then get that value. Here’s an example:
public class App {
public static void main(String[] args) {
Keyboard myKeyboard = new Keyboard();
myKeyboard.setFunctionKeys("f11, f10, f9");
myKeyboard.getFunctionKeys();
}
}
Here I’ve instantiated the class with an object called myKeyboard
. I then set the value by passing an argument to setFunctionKeys
method. The class then sets functionKeys
as that value. I then get the value by using the getter method: getFunctionKeys()
. Using this technique, I have encapsulated the variable against change by the user. If I wanted, I could entirely modify the meaning of the functionKeys string and users wouldn’t have to change anything about my code.
It’s pretty common with APIs to provide getter and setter methods to users. You don’t want to expose the inner workings of your classes. You just want to provide users with controls in order to use it.
About Tom Johnson
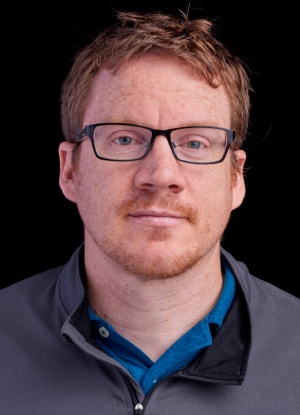
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.