Activity: Use methods with curl
Our sample weather API doesn’t allow you to use anything but a GET method, so for this exercise, to use other methods with curl, we’ll use the petstore API from Swagger. However, we won’t actually use the Swagger UI (which is something we’ll explore later). For now, we just need an API with which we can use to create, update, and delete content.
In this example, using the Petstore API, you’ll create a new pet, update the pet, get the pet’s ID, delete the pet, and then try to get the deleted pet.
- Create a new pet
- Update your pet
- Get your pet’s name by ID
- Delete your pet
- Understanding idempotent methods
- Import curl into Postman
- Export Postman to curl
Create a new pet
To create a pet, you have to pass a JSON message in the request body. Rather than trying to encode the JSON and pass it in the URL, you’ll store the JSON in a file and reference the file.
A lot of APIs require you to post requests containing JSON messages in the body. Request bodies are often how you configure a service. The list of JSON key-value pairs that the API accepts is called the “Model” in the Swagger UI display.
-
Insert the following into a text file. This information will be passed in the
-d
parameter of the curl request:{ "id": 123, "category": { "id": 123, "name": "test" }, "name": "fluffy", "photoUrls": [ "string" ], "tags": [ { "id": 0, "name": "string" } ], "status": "available" }
-
Change the first
id
value to another integer (a whole number in this case). Also, change the pet’s name offluffy
to something else.Use a unique ID and name that others aren’t likely to also use. Also, don’t begin your ID with the number 0.
- Save the file in a directory that you can conveniently access from your terminal, such as your user directory (on a Mac,
Users/YOURUSERNAME
— replaceYOURUSERNAME
with your actual user name on your computer). -
In your terminal, browse to the directory where you saved the mypet.json file. (Usually, the default directory is
Users/YOURUSERNAME
— hence the previous step.)If you’ve never browsed directories using the command line, here’s how you do it:
-
On a Mac, find your present working directory by typing
pwd
. Then move up a level by typing change directory:cd ../
. Move down a level by typingcd pets
, wherepets
is the name of the directory you want to move into. Typels
to list the contents of the directory. -
On Windows, look at the prompt path to see your current directory. Then move up a level by typing
cd ../
. Move down a level by typingcd pets
, wherepets
is the name of the directory you want to move into. Typedir
to list the contents of the current directory.
-
-
After your terminal or command prompt is in the same directory as your JSON file, create the new pet with the following curl request:
curl -X POST --header "Content-Type: application/json" --header "Accept: application/json" -d @mypet.json "https://petstore.swagger.io/v2/pet"
The
Content-Type
indicates the type of content submitted in the request body. TheAccept
indicates the type of content we will accept in the response.The response should look something like this:
{"id":51231236,"category":{"id":4,"name":"testexecution"},"name":"fluffernutter","photoUrls":["string"],"tags":[{"id":0,"name":"string"}],"status":"available"}
In the response, check to see that your pet’s name was returned.
Update your pet
Guess what, your pet hates its name! Change your pet’s name to something more formal using the update pet method.
- In the mypet.json file, change the pet’s name.
-
Use the
PUT
method instead ofPOST
to update the pet’s name (keep the same curl content otherwise):curl -X PUT --header "Content-Type: application/json" --header "Accept: application/json" -d @mypet.json "https://petstore.swagger.io/v2/pet"
Get your pet’s name by ID
Find your pet’s name by passing the ID into the /pet/{petID}
endpoint:
- In your mypet.json file, copy the first
id
value. -
Use this curl command to get information about that pet ID, replacing
51231236
with your pet ID.curl -X GET --header "Accept: application/json" "https://petstore.swagger.io/v2/pet/51231236"
The response contains your pet’s name and other information:
{"id":51231236,"category":{"id":4,"name":"test"},"name":"mr. fluffernutter","photoUrls":["string"],"tags":[{"id":0,"name":"string"}],"status":"available"}
You can format the JSON by pasting it into a JSON formatting tool:
{ "id": 51231236, "category": { "id": 4, "name": "test" }, "name": "mr. fluffernutter", "photoUrls": [ "string" ], "tags": [ { "id": 0, "name": "string" } ], "status": "available" }
Delete your pet
Unfortunately, your pet has died. It’s time to delete your pet from the pet registry.
-
Use the DELETE method to remove your pet. Replace
5123123
with your pet ID:curl -X DELETE --header "Accept: application/json" "https://petstore.swagger.io/v2/pet/5123123"
-
Now check to make sure your pet is removed. Use a GET request to look for your pet with that ID:
curl -X GET --header "Accept: application/json" "https://petstore.swagger.io/v2/pet/5123123"
You should see this error message:
{"code":1,"type":"error","message":"Pet not found"}
This example allowed you to see how you can work with curl to create, read, update, and delete resources. These four operations are referred to as CRUD and are common to almost every programming language.
Although Postman is probably easier to use, curl lends itself to power-level usage. Quality assurance teams often construct advanced test scenarios that iterate through a lot of curl requests.
Understanding idempotent methods
One concept important to understand with HTTP methods is “idempotency.” Roy Fielding defines idempotency as follows:
A request method is considered “idempotent” if the intended effect on the server of multiple identical requests with that method is the same as the effect for a single such request. Of the request methods defined by this specification, PUT, DELETE, and safe request methods are idempotent” (RFC 7231, 4.2.2.
In other words, with idempotent methods, you can run them multiple times without multiplying the results. Idempotent methods include GET, PUT, and DELETE, while POST is not (see 8.1.3 for a more detailed list).
Todd Fredrich explains idempotency by comparing it to a pregnant cow. Let’s say you bring over a bull to get a cow pregnant. Even if the bull and cow mate multiple times, the result will be just one pregnancy, not a pregnancy for each mating session.
Import curl into Postman
You can import curl commands into Postman by doing the following:
- Open a new tab in Postman and click the Import button in the upper-left corner.
-
Select Paste Raw Text and insert your curl command:
curl -X GET --header "Accept: application/json" "https://petstore.swagger.io/v2/pet/5123123"
Make sure you don’t have any extra spaces at the beginning.
- Click Import.
- Close the dialog box.
- Click Send. (If you deleted your pet, you will see the same “Pet not found” error message as before.)
Export Postman to curl
You can also export Postman to curl by doing the following:
- If desired, select one of your OpenWeatherMap API requests in Postman.
-
Click the Code button (it’s right below Save).
- Select curl from the drop-down menu.
-
Copy the code snippet.
curl -X GET \ 'https://api.openweathermap.org/data/2.5/weather?lat=37.3565982&lon=-121.9689848&units=imperial&appid=APIKEY'
In place of
APIKEY
you should see your actual API key. - Remove the backslashes and line breaks. If you’re on Windows, change the single quotes to double quotes.
-
Insert the curl command into your terminal and observe the result.
curl -X GET "https://api.openweathermap.org/data/2.5/weather?lat=37.3565982&lon=-121.9689848&units=imperial&appid=APIKEY"
Through Postman’s Import and Code functionality, you can easily switch between Postman and curl.
About Tom Johnson
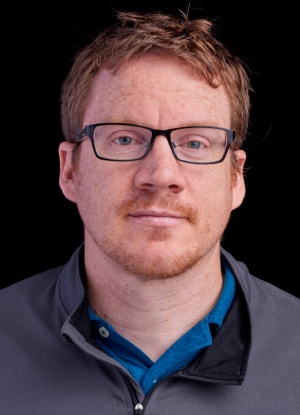
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.
20/166 pages complete. Only 146 more pages to go.