Dive into dot notation
In the previous topic, Access and print a specific JSON value, you accessed and printed a specific JSON value to the page. Let’s dive into dot notation a little more since understanding how to access the right JSON value you want is necessary to make use of the response.
- Dot notation
- Use square brackets to access the values in an array
- Exercise with dot notation
- Showing wind conditions on the page
- Next section
Dot notation
You use a dot after the object name to access its properties. For example, suppose you have an object called data
:
"data": {
"name": "Tom"
}
To access Tom
, you would use data.name
.
Note the different levels of nesting so you can trace back the appropriate objects and access the information you want. You access each level down through the object name followed by a dot.
Use square brackets to access the values in an array
To access a value in an array, you use square brackets followed by the position number. For example, suppose you have the following array:
"data" : {
"items": ["ball", "bat", "glove"]
}
To access glove, you would use data.items[2]
.
glove
is the third item in the array. You can’t access an item directly in an array by the item’s name — only by its position. Usually, programmers loop through an array and pull out values that match.
With most programming languages, you usually start counting at 0
, not 1
.
Exercise with dot notation
In this activity, you’ll practice accessing different values through dot notation.
-
Create a new file in your text editor and insert the following into it:
<!DOCTYPE html> <html> <head> <script src="https://code.jquery.com/jquery-2.1.1.min.js"></script> <meta charset="utf-8"> <title>JSON dot notation practice</title> <script> $( document ).ready(function() { var john = { "hair": "brown", "eyes": "green", "shoes": { "brand": "nike", "type": "basketball" }, "favcolors": [ "azure", "goldenrod" ], "children": [ { "child1": "Sarah", "age": 2 }, { "child2": "Jimmy", "age": 5 } ] } var sarahjson = john.children[0].child1; var greenjson = john.children[0].child1; var nikejson = john.children[0].child1; var goldenrodjson = john.children[0].child1; var jimmyjson = john.children[0].child1; $("#sarah").append(sarahjson); $("#green").append(greenjson); $("#nike").append(nikejson); $("#goldenrod").append(goldenrodjson); $("#jimmy").append(jimmyjson); }); </script> </head> <body> <div id="sarah">Sarah: </div> <div id="green">green: </div> <div id="nike">nike: </div> <div id="goldenrod">goldenrod: </div> <div id="jimmy">Jimmy: </div> </body> </html>
Here we have a JSON object defined as a variable named
john
. (Usually, APIs retrieve the response through a URL request, but for practice here, we’re just defining the object locally.)If you view the page in your browser, you’ll see the page says “Sarah” for each item because we’re accessing this value:
john.children[0].child1
for each item.var sarahjson = john.children[0].child1; var greenjson = john.children[0].child1; var nikejson = john.children[0].child1; var goldenrodjson = john.children[0].child1; var jimmyjson = john.children[0].child1;
-
Change
john.children[0].child1
to display the correct values for each item. For example, the wordgreen
should appear at the ID tag calledgreen
.
You can view the correct page here: https://idratherbewriting.com/assets/files/dot-notation-practice.html. This page also shows the answers printed.
Showing wind conditions on the page
At the beginning of the section on Using an API like a developer, I showed an example of embedding the wind speed and other details on a website. Now let’s revisit this code example and see how it’s put together.
Copy the following code into a basic HTML file:
<!DOCTYPE html>
<html>
<head>
<script src="https://code.jquery.com/jquery-2.1.1.min.js"></script>
<meta charset="utf-8">
<link rel="stylesheet" href='https://maxcdn.bootstrapcdn.com/bootstrap/3.3.4/css/bootstrap.min.css' rel='stylesheet' type='text/css'>
<title>OpenWeatherMap Integration</title>
<style>
#wind_direction, #wind_speed, #wind_speed_unit, #wind_degree_unit, #weather_conditions, #main_temp_unit, #main_temp {color: red; font-weight: bold;}
body {margin:20px;}
</style>
</head>
<body>
<script>
function checkWind() {
var settings = {
"async": true,
"crossDomain": true,
"dataType": "json",
"url": "https://api.openweathermap.org/data/2.5/weather?zip=95050,us&appid=APIKEY&units=imperial",
"method": "GET"
}
$.ajax(settings)
.done(function (response) {
console.log(response);
$("#wind_speed").append (response.wind.speed);
$("#wind_direction").append (response.wind.deg);
$("#main_temp").append (response.main.temp);
$("#weather_conditions").append (response.weather[0].main);
$("#wind_speed_unit").append (" MPH");
$("#wind_degree_unit").append (" degrees");
$("#main_temp_unit").append (" F");
});
}
</script>
<button type="button" onclick="checkWind()" class="btn btn-danger weatherbutton">Check wind conditions</button>
<h2>Wind conditions for Santa Clara</h2>
<span><b>Temperature: </b></span><span id="main_temp"></span><span id="main_temp_unit"></span><br/>
<span><b>Wind speed: </b></span><span id="wind_speed"></span> <span id="wind_speed_unit"></span><br/>
<span><b>Wind direction: </b></span><span id="wind_direction"></span><span id="wind_degree_unit"></span><br/>
<span><b>Current conditions: </b></span><span id="weather_conditions"></span>
</body>
</html>
(In the above code, replace APIKEY
with your actual API key.)
A few things are different here, but it’s essentially the same code as you created in the Access and print a specific JSON value. Here’s what’s different:
- Rather than running the
ajax
method on page load, theajax
method is wrapped inside a function calledcheckWind
. When the web page’s button is clicked, theonclick
method fires thecheckWind()
function. - When the
checkWind
function runs, the values for temperature, wind speed, wind direction, and current conditions are written to several ID tags on the page.
When you load the page and click the button, the following should appear:
You can view the file idratherbewriting.com/assets/files/wind-openweathermap.html.
Next section
As you’ve progressed through the exercises for using an API like a developer, you’ve gained a high-level understanding of how REST APIs work, what information developers need, how they might use an API, how they make requests, evaluate responses, and other details.
With this background, it’s time to switch gears and put on your technical writing hat. In the next section, Documenting endpoints, you’ll assume the task of documenting a new endpoint that was added to a weather API. You’ll learn the essential sections in endpoint reference documentation, the terminology to use, and formatting conventions for API reference information.
About Tom Johnson
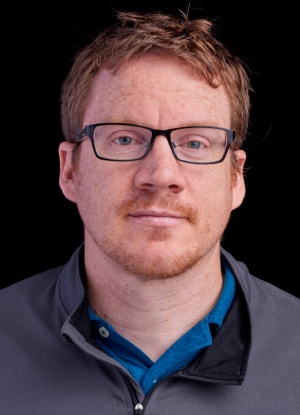
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.
24/165 pages complete. Only 141 more pages to go.