Access and print a specific JSON value
This tutorial continues from the previous topic, Inspect the JSON from the response payload. In the sample page where you logged the weather
response to the JS Console, the REST response information didn’t appear on the page. It only appeared in the JS Console. You need to use dot notation and JavaScript to access the JSON values you want. In this tutorial, you’ll use JavaScript to print some of the response to the page.
Note that this section will use a little bit of JavaScript. Depending on your role, you might not use this code much in your documentation, but it’s important to know anyway.
- Getting a specific property from a JSON response object
- Printing a JSON value to the page
- Get the value from an array
- More exercises
Getting a specific property from a JSON response object
JSON wouldn’t be very useful if you always had to print out the entire response. Instead, you select the exact property you want and pull that out through dot notation. The dot (.
) after response
(the name of the JSON payload, as defined arbitrarily in the jQuery AJAX function) is how you access the values you want from the JSON object.
As an example, this is the full response from the request made previously:
{
"coord": {
"lon": -121.95,
"lat": 37.35
},
"weather": [
{
"id": 802,
"main": "Clouds",
"description": "scattered clouds",
"icon": "03d"
}
],
"base": "stations",
"main": {
"temp": 68.34,
"pressure": 1014,
"humidity": 73,
"temp_min": 63,
"temp_max": 72
},
"visibility": 16093,
"wind": {
"speed": 3.36
},
"clouds": {
"all": 40
},
"dt": 1566664878,
"sys": {
"type": 1,
"id": 5122,
"message": 0.0106,
"country": "US",
"sunrise": 1566653501,
"sunset": 1566701346
},
"timezone": -25200,
"id": 0,
"name": "Santa Clara",
"cod": 200
}
In our scenario (creating a biking app), we want to pull out the wind speed part of the JSON response. Here’s the dot notation you use:
response.wind.speed
To pull out the wind speed element from the JSON response and print it to the JavaScript Console, add this to your code sample (which you created in the previous tutorial), right below the console.log(response)
line:
console.log("wind speed: " + response.wind.speed);
Your code should look like this:
$.ajax(settings).done(function (response) {
console.log(response);
console.log("wind speed: " + response.wind.speed);
});
Refresh your Chrome browser and see the information that appears in the console:
wind speed: 13.87
Printing a JSON value to the page
Let’s say you wanted to print part of the JSON (the wind speed data) to the page, not just the console. (By “print,” I mean make the value appear on the page, not send it to a printer.) Printing the value involves a little bit of JavaScript (or jQuery to make it easier).
I’m assuming you’re starting with the same code from the previous tutorial. That code looks like this:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<title>Sample Page</title>
<script>
var settings = {
"async": true,
"crossDomain": true,
"url": "https://api.openweathermap.org/data/2.5/weather?zip=95050&appid=APIKEY&units=imperial",
"method": "GET"
}
$.ajax(settings).done(function (response) {
console.log(response);
});
</script>
</head>
<body>
<h1>Sample Page</h1>
</body>
</html>
(In the above code, replace APIKEY
with your actual API key.)
To print a specific property from the response to the page,
-
Add the following inside the
ajax
function:$.ajax(settings).done(function (response) { console.log(response); var content = response.wind.speed; $("#windSpeed").append(content); });
Your code should look as follows:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <title>Sample Page</title> <script> var settings = { "async": true, "crossDomain": true, "url": "https://api.openweathermap.org/data/2.5/weather?zip=95050&appid=APIKEY&units=imperial", "method": "GET" } $.ajax(settings).done(function (response) { console.log(response); var content = response.wind.speed; $("#windSpeed").append(content); }); </script> </head> <body> <h1>Sample Page</h1> <div id="windSpeed">Wind speed: </div> </body> </html>
(In the above code, replace
APIKEY
with your actual API key.) -
Refresh the page and you will see the wind speed printed to the page. Here’s an example with both wind speed and weather conditions.
Here’s what we changed:
Inside the tags of the AJAX done
method, we pulled out the value we wanted into a variable, like this:
var content = response.wind.speed;
Then we added a named element to the body of the page, like this:
<div id="windSpeed">Wind speed: </div>
We used the jQuery append
method to append the content
variable to the element with the windSpeed
ID on the page:
$("#windSpeed").append(content);
This code says to find the element with the ID windSpeed
and add the content
variable after it.
Get the value from an array
In the previous section, you retrieved a value from a JSON object. Now let’s get a value from an array. Let’s get the main
property from the weather
array in the response. Here’s what the JSON array looks like:
{
"weather": [
{
"id": 801,
"main": "Clouds",
"description": "few clouds",
"icon": "02d"
}
]
]
}
Remember that brackets signify an array. Inside the weather
array is an unnamed object. To get the main
element from this array, you would use the following dot notation:
response.weather[0].main
Then you would follow the same pattern as before to print it to the page. While objects allow you to get a specific property, arrays require you to select the position in the list that you want.
Here’s the code from the sample page:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<title>Sample Page</title>
<script>
var settings = {
"async": true,
"crossDomain": true,
"url": "https://api.openweathermap.org/data/2.5/weather?zip=95050&appid=APIKEY&units=imperial",
"method": "GET"
}
$.ajax(settings).done(function (response) {
console.log(response);
var content = response.wind.speed;
$("#windSpeed").append(content);
var currentWeather = response.weather[0].main;
$("#currentWeather").append(currentWeather);
});
</script>
</head>
<body>
<h1>Sample Page</h1>
<div id="windSpeed">Wind speed: </div>
<div id="currentWeather">Current weather conditions: </div>
</body>
</html>
(In the above code, replace APIKEY
with your actual API key.)
More exercises
If you’d like to follow some more exercises that involve calling REST APIs, accessing specific values, and printing the values to the page, see the following topics in the Glossary and resources section:
- Get event information using the Eventbrite API
- Flickr example: Retrieve a Flickr gallery
- Get wind speed using the Aeris Weather API
About Tom Johnson
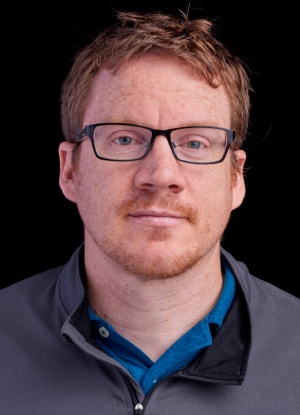
I'm an API technical writer based in the Seattle area. On this blog, I write about topics related to technical writing and communication — such as software documentation, API documentation, AI, information architecture, content strategy, writing processes, plain language, tech comm careers, and more. Check out my API documentation course if you're looking for more info about documenting APIs. Or see my posts on AI and AI course section for more on the latest in AI and tech comm.
If you're a technical writer and want to keep on top of the latest trends in the tech comm, be sure to subscribe to email updates below. You can also learn more about me or contact me. Finally, note that the opinions I express on my blog are my own points of view, not that of my employer.
23/166 pages complete. Only 143 more pages to go.